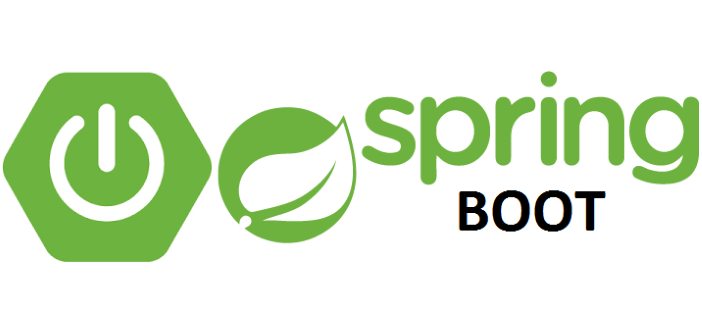
1. SpringBoot快速入门
1.1 Quickstart
- pom.xml
1 | <parent> |
- 主程序
1 |
|
- 业务逻辑
1 |
|
- 默认包结构
- 主程序同包或主程序子包下的类
- 使用
@SpringBootApplication(scanBasePackages="indi.pancras")
指定 - 使用
@ComponentScan("indi.pancras")
指定扫面路径
1.2 配置绑定
- Car.java
1 |
|
- application.properties
1 | BYD = |
2. SpringBoot的两大机制
2.1 依赖管理
- 父项目做依赖管理
1 | <parent> |
- 开发场景导入starter场景启动器
1 | <dependency> |
2.2 自动配置
- 自动配好Tomcat
- 引入Tomcat依赖
- 自动配好SpringMVC
- 自动配好web常见功能:如字符编码问题
- 默认的包结构
- 各种配置拥有默认值
- 按需加载所有自动配置项
- …
3. SpringBoot功能
3.1 组件添加
- @Configuration
1 |
|
Full 模式和 Lite 模式
- 组件无依赖:采用Lite模式,启动快
- 组件有依赖:采用Full模式,多了一些判断步骤
@Import
- 向容器中加入组件,默认是全类名
@Conditional
- 条件装配
@ImportResource
- 导入xml中的bean
3.2 配置绑定
将properties中的配置绑定到配置类中
@ConfigurationProperties+@Component
@Component @ConfigurationProperties(prefix = "mycar") public class Car { private String brand; private Integer price; } //mycar.brand=BYD //mycar.price=100000
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
+ @EnableConfigurationProperties
+ ```java
@Configuration
@EnableConfigurationProperties(Car.class)// 开启Car的属性配置
public class MyConfig {
}
@ConfigurationProperties(prefix = "car")
@Data
@ToString
@AllArgsConstructor
@NoArgsConstructor
public class Car {
private String brand;
private Integer price;
}
3.3 最佳实践
- 引入场景依赖
- 修改配置项
- 替换部分组件
4. Web开发
4.1 应用配置项
- 静态资源的访问
1 | spring: |